GAMES LESSON Twenty-three: Adding Background to Terrain Loop
A background picture is a nice touch to add to a game. It is especially nice
if as the player moves, the background also moves. BUT it is even nicer if
the background moves at a rate which is slower than the player (who is in
the foreground) since this makes it seem like the background is farther
away.
Here's the background image in its entirety. Obviously you can only see a
small portion of it at a time in the applet.
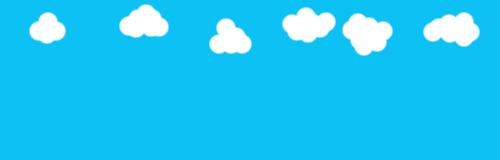
This applet is exactly the same as the applet used in the previous lesson
except that the background image is used instead of the tiles which were
used for the sky. A few variables were added and a few discarded and a few
details are different, but essentially it's the same applet. You should look
it over carefully and compare it to the previous applet to see where the
differences occur.
One major problem is that the background on the player tile is not the same
color as the background image. If we had a varied background image (with a
much more interesting background scene) it would be impossible to create a
tile with a matching background unless the background of the tile were
transparent. This is something we deal with in the next lesson.
import java.awt.*;
import java.applet.*;
import java.awt.event.*;
public class TERR2 extends Applet{
Dimension d;
//brick=0, sky=1, guy=2
int[][] map = {
{ 1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1},
{ 1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1},
{ 1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1},
{ 1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,0,1,1,1,1,1,1,1,1,1},
{ 1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,0,0,0,1,1,1,1,1,1,1,1},
{ 1,1,1,1,1,0,1,1,1,1,1,1,1,1,1,1,0,0,0,0,0,1,1,1,1,1,1,1},
{ 1,1,1,1,0,0,0,1,1,1,1,2,1,1,1,0,0,0,0,0,0,0,1,1,1,1,1,1},
{ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0},
};
//28x8
int xguy=11;
int yguy=6;
int xedge = xguy - 5;
int bgx=-100;
int taps;
Image bg, brick, guy;
Image offI;
public void init(){
d = getSize();
offI=createImage(d.width,d.height);
brick = getImage(getDocumentBase(), "brick.jpg");
bg = getImage(getDocumentBase(), "bg.jpg");
guy = getImage(getDocumentBase(), "guy.jpg");
requestFocus();
this.addKeyListener(new KeyAdapter(){
public void keyPressed(KeyEvent k) {
if(k.getKeyCode() == KeyEvent.VK_LEFT){
if(taps%2==0) bgx++;
if(xguy>0){
map[yguy][xguy]=1;
xguy--;
if(map[yguy][xguy]==1) map[yguy][xguy]=2;
else{
yguy--;
map[yguy][xguy]=2;
}
}
else{
map[yguy][xguy]=1;
xguy=27;
if(map[yguy][xguy]==1) map[yguy][xguy]=2;
else{
yguy--;
map[yguy][xguy]=2;
}
}
}
else if(k.getKeyCode() == KeyEvent.VK_RIGHT){
if(taps%2==0) bgx--;
map[yguy][xguy]=1;
xguy++;
xguy%=28;
if(map[yguy][xguy]==1) map[yguy][xguy]=2;
else{
yguy--;
map[yguy][xguy]=2;
}
}
if(bgx>0) bgx=0;
if(bgx<-300) bgx=-300;
if(map[yguy+1][xguy]==1){
map[yguy][xguy]=1;
yguy++;
map[yguy][xguy]=2;
}
xedge = xguy-5;
if(xedge<0) xedge=28+xedge;
taps++;
repaint();
}
});
}
public void update(Graphics g){
paint(g);
}
public void paint(Graphics g){
Graphics offG = offI.getGraphics();
offG.setColor(Color.black);
offG.drawImage(bg, bgx, 0, this);
int hori=0;
for(int y=0; y
ASSIGNMENT: